How React Works
The elegant way react understands our code
How react works?
Have your ever wondered how react works? How does it parse the data you code? How does it convert the jsx into desired elements and render them. The only thing we know for sure is that react app is compiled to basic html, css and js files. But what is the internal mechanism. Lets discuss this.
The beauty of react is that it allows to 'write html' in the code directly. Why inverted commas? Because, technically, it is not html but rather html-looking js or more commonly known as JSX (Javascript XML), a syntex extension to javascript element.
Lets say you want to create a simple component
const buttonTitle = "Submit Me";
return (
<button className="btn btn-blue">
<span>{buttonTitle}</span>
</button>
);
React would take this JSX and translate into elements of react which is nothing but an object representing this JSX like this below:
{
type: 'button',
props: {
className: 'btn btn-blue',
children: {
type: 'span',
children: 'Submit Me'
}
}
}
And this object is how react sees the code that is coded in jsx above. It takes each node and convert it onto a plain object. This plain object is known as react element.
The object that is created mainly consist of two major attributes:
- types: it defines the type of nodes, e.g. button or div or span
- props: all the properties that component received e.g. classNames, children, id.
This conversion process is started at top and is repeated until the last node is converted. The result is a one gaint object which is also known as tree or more specifically DOM tree. These objects does not exist in reality i.e. they dont refer to anything on screen, they are just objects but the important point is that these objects are easy to traverse.
Lets look into another example:
const logout = () => {
return (
<div>
<p>Are you sure?</p>
<SubmitBtn color="blue">Yes, please</SubmitBtn>
</div>
);
};
This would complie into:
{
type: "div",
props: [
{
type: 'p',
props: {
children: 'Are you sure?'
}
},
{
type: 'SubmitBtn',
props: {
color: 'blue',
children: 'Yes, please'
}
}
]
}
As can be seem in example above, it does not matter whether it is a native HTML element or user-created custom element, it becomes part of the object tree. This keeps components decoupled and maintain relationship through composition. When react sees an element with a function type like the submit button, it will know to ask that component what element it renders to with the given props. So React will ask Submit button again what it renders to and it will transform that into an element. React will keep repeating this process until it knows the underlying DOM tag elements for every component on the page. Once React finishes the process of identifying all user defined components from the tree of elements, it will convert them into DOM elements. The result is what is generally known as the virtual DOM [1].
A change in UI
What happens when there is a change in ui? Liken there is a change triggered via some button interactions or via state update by useState hook or maybe UI is changed as API brings in a new data? All these would lead to a ui change or more specifically a change in object tree [1].
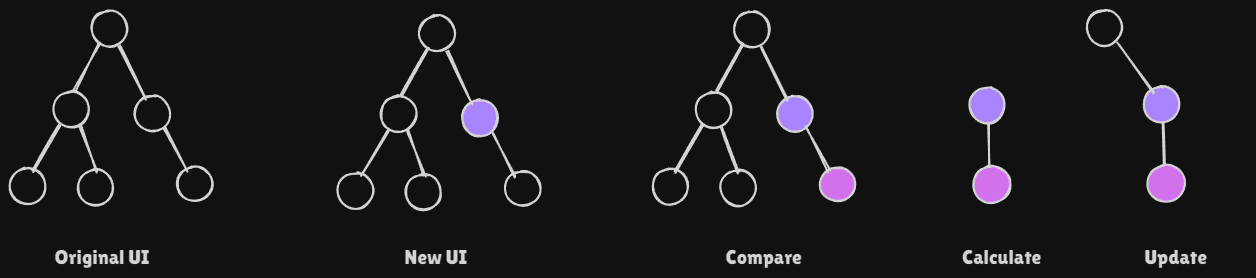
First, React will take all your JSX and produce a new UI representation as a tree of elements. Second, it will compare it with the previous representation that is kept in memory. Third, it will calculate the difference in a tree, recall that since each node in the tree is a JavaScript object, this diffing operation is very fast. And finally based on that difference, it will apply the minimum number of changes to the underlying dom nodes in order to process the update, and that's it.
React Composition Model
React works on composition model in comparison to inheritance model unlike other frameworks [2]. React composition model consist of two main features:
- Containment
- Specialization
Before discussing above two terms, the main term which is central to above two is 'children prop'. All components have children props and is central to composition model.
In many instances, it is not possible to know children in advance. This provides the flexibility to code anything within that children prop. E.g a dialog box may have dynamic children but dialog box may not know in advance what the children would be if it is not hard-coded. This is containment. If a component is derivative of another main generic component and present the specialized component, this depicts Specialization. Lets understand it via an example:
const App = () => {
const Alert = ({ children }) => <div>{children}</div>;
const SimpleButton = ({ value, color }) => (
<button style={{ backgroundColor: color }}>{value}</button>
);
const RedButton = ({ value }) => (
<SimpleButton style={{ backgroundColor: "red" }}>{value}</SimpleButton>
);
return (
<main>
<Alert>
Are you sure you want to logout?
<RedButton value="yes" />
<SimpleButton value="no" color="grey" />
</Alert>
</main>
);
};
The alert component depicts containment as any children can be coded here. While RedButton is a special case of SimpleButton component.
References
[1] https://www.coursera.org/learn/advanced-react/lecture/LcYik/jsx-components-and-elements
[2] https://legacy.reactjs.org/docs/composition-vs-inheritance.html